When creating news lists, blog cards, or product grids, you often need to display links to detailed pages using thumbnails and only the beginning of the content. In such cases, implementing ellipsis to truncate the text is often required. This article explains how to achieve this using both JavaScript and CSS.
Using JavaScript to Add Ellipsis
With JavaScript, you can control the number of characters displayed and add an ellipsis if the text exceeds the specified length.
Example using slice
The slice
method allows you to extract a portion of a string. The first argument specifies the start index (starting from 0), and the second argument specifies the end index (optional).
For example, in the code below, extract the first 30 characters from a string and append “…” :
const paragraph = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.';
// Extract the first 30 characters and append "..."
<p>{paragraph.slice(0, 30)}...</p>
// Output: Lorem ipsum dolor sit amet, co...
While this approach is simple to implement, it has a drawback: even if the text is shorter than 30 characters, it will still add "..."
. To address this, you’ll need to create a function to conditionally add an ellipsis based on the text length.
Example using Custom Hook (React.js / Next.js)
In React.js or Next.js, you can encapsulate reusable logic in a custom hook. This keeps your code clean and avoids duplication across your components.
import { useMemo } from "react";
// paragraph: the text to display
// textNum: the maximum number of characters to show
export const useTextCount = (paragraph, textNum) => {
const truncatedText = useMemo(() => {
return paragraph.length > textNum
? paragraph.slice(0, textNum) + "…" // Example: "Lorem ipsum dol..."
: paragraph; // Return the original string if it's shorter than textNum
}, [paragraph, textNum]);
return truncatedText;
};
Applying the Hook.
// Pass the text and the maximum character count as arguments:
<p>{useTextCount(item.content, 100)}</p>
Here’s an example that integrates the hook into a React component, using TailwindCSS for styling. TailwindCSS makes it easy to apply styles, which is particularly helpful for creating responsive UI.
import newsData from "./constant/news.json";
import { useTextCount } from "./hooks/useTextCount";
function App() {
return (
<main className="flex flex-col items-center gap-8 py-16 max-w-[1280px] mx-auto">
<h1 className="text-4xl font-bold">News</h1>
<div className="w-full grid grid-cols-3 gap-6">
{newsData.news.map((item) => (
<a
href={`news/${item.id}`}
className="rounded-lg shadow-md border border-gray-600 bg-gray-50"
>
<div className="w-full">
<img
src={item.img}
alt=""
className="rounded-t-lg max-h-[300px]"
/>
</div>
<div className="p-3 flex flex-col gap-2">
<span className="text-sm text-gray-400">{item.date}</span>
<h2 className="font-bold">{item.title}</h2>
<p>{useTextCount(item.content, 30)}</p>
<span className="text-sm text-right">Read more</span>
</div>
</a>
))}
</div>
</main>
);
}
export default App;
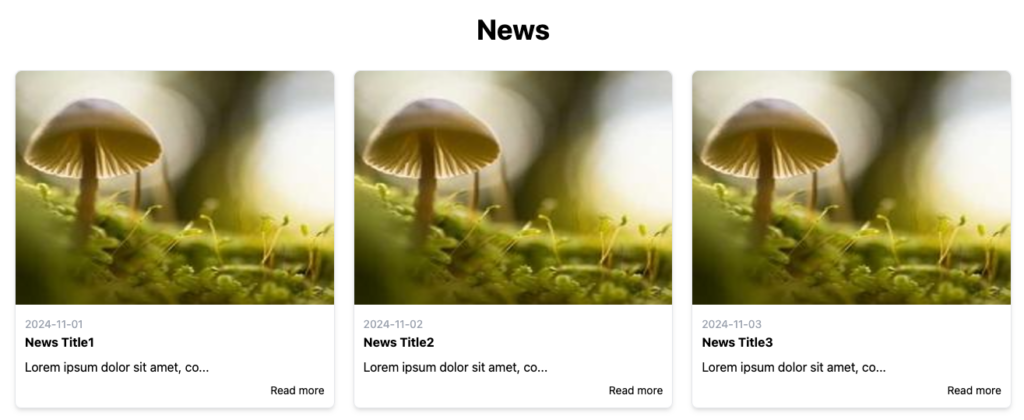
For simpler use cases, you can stick to the slice
method. For more complex scenarios or reusable logic in React.js/Next.js environments, consider using the custom hook approach.
Using CSS to Add Ellipsis
With CSS, you can automatically add ellipsis to truncated text by applying specific styles to the container. The great thing about this approach is that it adjusts dynamically based on the content width, making it ideal for responsive designs.
Example using TailwindCSS
TailwindCSS has a built-in truncate
class that makes this very simple. However, note that this class works only for single-line text. For multi-line truncation, you’ll need to use custom CSS.
<p class="trunscate">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
This is equivalent to the following CSS:
.truncate {
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
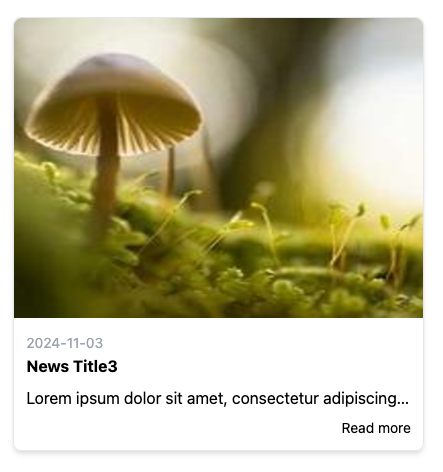
Example with Multi-Line Truncation in CSS
To display an ellipsis after a specific number of lines, you can use the -webkit-line-clamp
property. This works in modern browsers like Chrome, Edge, and Safari but is not supported in Internet Explorer.
<p class="truncate-multiple">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat</p>
.truncate-multiple {
display: -webkit-box;
-webkit-line-clamp: 3; /* Specify the number of lines */
-webkit-box-orient: vertical;
overflow: hidden;
}
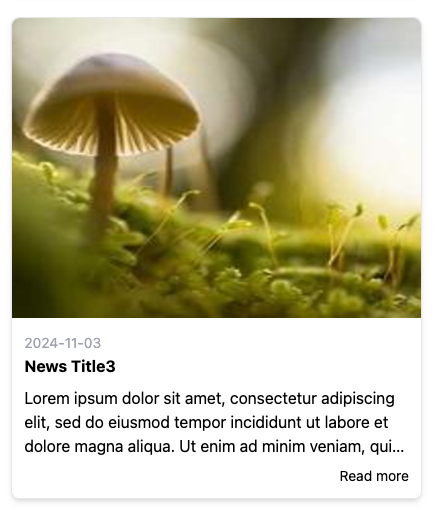
Conclusion
We’ve covered how to implement ellipsis “…” using JavaScript and CSS.
- If you need to control text length for specific use cases or multiple components, use JavaScript with a custom function or hook.
- If you want a responsive, width-based solution that looks clean and adapts to different screen sizes, CSS is the way to go.
Choose the method that best fits your needs and project requirements!